Store a null-terminated string
- Allocates a new varying-length structure.
The mi_string_to_lvarchar() function allocates the varying-length descriptor, setting the data length and data pointer appropriately. Both the data length and the size of the data portion are the length of the null-terminated string without its null terminator.
Server only: The mi_string_to_lvarchar() function allocates the varying-length structure that it creates with the current memory duration. - Copies the data of the null-terminated string into the newly allocated
data portion.
The mi_string_to_lvarchar() function does not copy the null terminator of the string.
- Returns a pointer to the newly allocated varying-length structure.
char *local_var;
mi_larchar *lvarch;
...
/* Allocate memory for null-terminated string */
local_var = (char *)mi_alloc(200);
/* Create the varying-length data to store */
sprintf(local_var, "%s %s %s", "A varying-length structure ",
"stores data in a data portion, which is separate from ",
"the varying-length structure.");
/* Store the null-terminated string as varying-length data */
lvarch = mi_string_to_lvarchar(local_var);
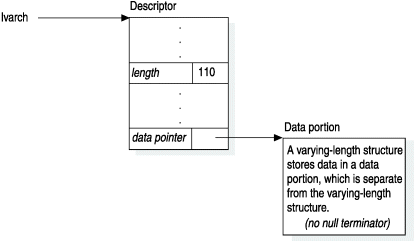
The lvarch varying-length structure in Figure 1 has a data length of 110. The null terminator is not included in the data length because the mi_string_to_lvarchar() function does not copy the null terminator into the data portion.
- Increment the data length accordingly and save it in the varying-length descriptor with the mi_set_varlen() function.
- Copy the data, including the null terminator, into the varying-length
structure with the mi_set_vardata() or mi_set_vardata_align() function.
These functions copy in the null terminator because the data length includes the null-terminator byte in its count. These functions assume that the data portion is large enough to hold the string and any null terminator.
#define TEXT_LENGTH 200
...
mi_lvarchar *lvarch;
char *var_text;
mi_integer var_len;
...
/* Allocate memory for null-terminated string */
var_text = (char *)mi_alloc(TEXT_LENGTH);
/* Create the varying-length data to store */
sprintf(var_text, "%s %s %s", "A varying-length structure ",
"stores data in a data portion, which is separate from ",
"the varying-length structure.");
var_len = stleng(var_text) + 1;
/* Allocate a varying-length structure to hold the
* null-terminated string (with its null terminator)
*/
lvarch = mi_new_var(var_len);
/* Copy the number of bytes that the data length specifies
* (which includes the null terminator) into the
* varying-length structure
*/
mi_set_vardata(lvarch, var_text);
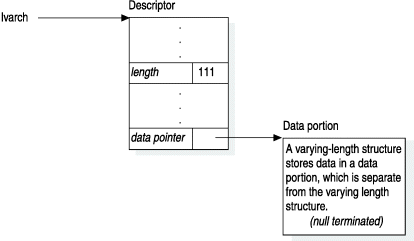